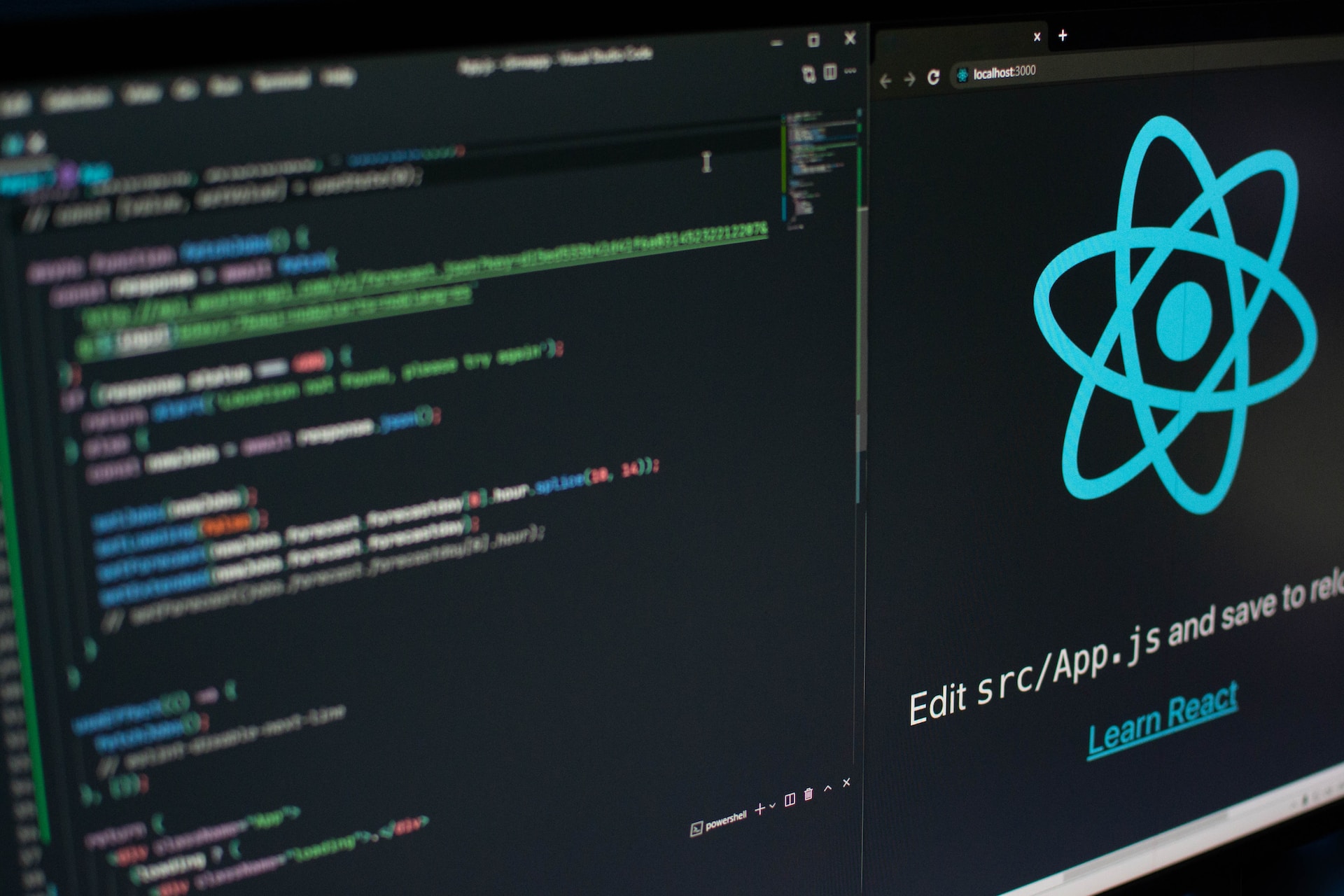
Getting Started with React - Create your first app
React is a popular JavaScript library for building user interfaces, and it's widely used for developing modern, single-page applications. Here's a step-by-step guide to help you get started with React:
1. Set Up Your Development Environment:
Node.js and npm:
Ensure you have Node.js installed on your machine. npm (Node Package Manager) comes with Node.js.
Create React App:
You can use Create React App, a tool that sets up a new React project with a good default configuration. Open your terminal and run:
npx create-react-app my-react-app
Replace "my-react-app" with the name you prefer for your project.
2. Project Structure:
Navigate into your project directory:
cd my-react-app
Explore the project structure. The main files you'll work with are located in the src
folder.
3. Understanding Components:
React is based on the concept of components. Components are reusable, self-contained building blocks for your UI. There are two types of components: functional and class components.
Functional Components (with Hooks):
import React, { useState } from 'react';
function MyComponent() {
const [count, setCount] = useState(0);
return (
<div>
<p>Count: {count}</p>
<button onClick={() => setCount(count + 1)}>Increment</button>
</div>
);
}
export default MyComponent;
Class Components:
import React, { Component } from 'react';
class MyComponent extends Component {
constructor(props) {
super(props);
this.state = {
count: 0,
};
}
render() {
return (
<div>
<p>Count: {this.state.count}</p>
<button onClick={() => this.setState({ count: this.state.count + 1 })}>
Increment
</button>
</div>
);
}
}
export default MyComponent;
4. JSX and Rendering:
JSX is a syntax extension for JavaScript that looks similar to XML or HTML. It's used with React to describe what the UI should look like.
import React from 'react';
function App() {
return (
<div>
<h1>Hello, React!</h1>
<MyComponent />
</div>
);
}
export default App;
5. Running Your App:
Back in your terminal, run:
npm start
This command starts the development server, and you can view your app at http://localhost:3000
in your browser.
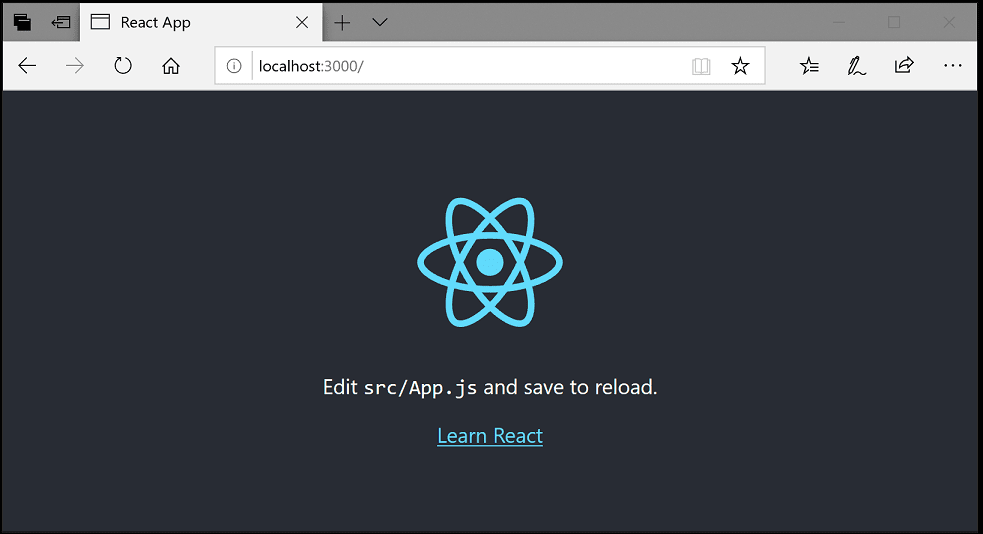
Application structure
create-react-app gives us everything we need to develop a React application. Its initial file structure looks like this:
my-react-app
├── README.md
├── node_modules
├── package.json
├── package-lock.json
├── .gitignore
├── public
│ ├── favicon.ico
│ ├── index.html
│ ├── logo192.png
│ ├── logo512.png
│ ├── manifest.json
│ └── robots.txt
└── src
├── App.css
├── App.js
├── App.test.js
├── index.css
├── index.js
├── logo.svg
├── reportWebVitals.js
└── setupTests.js
6. Learn and Experiment:
Explore the React documentation (https://reactjs.org/) to deepen your understanding. Experiment with creating more components, using state and props, and incorporating other React concepts.
7. Tools and Extensions:
Consider using tools like React DevTools and VS Code extensions for React to enhance your development experience.
That's a quick start to React! As you become more comfortable, you can explore state management with Redux, routing with React Router, and integration with backend services. Happy coding!
Comments